Button
A button is component that the user can press to trigger an action.
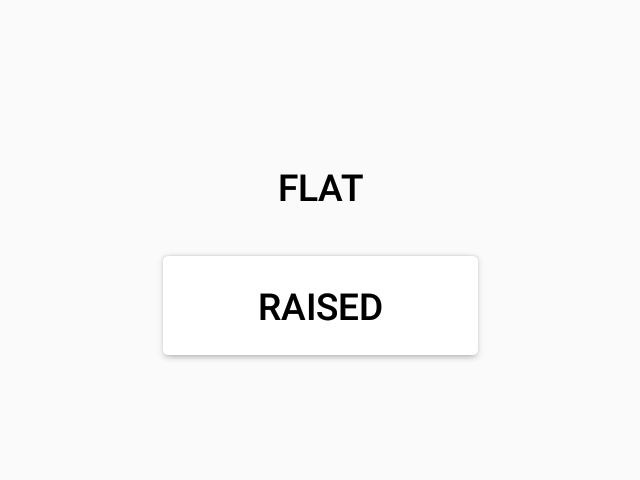
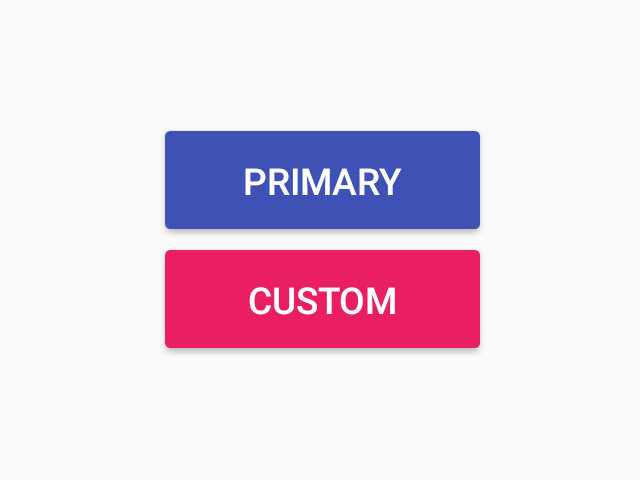
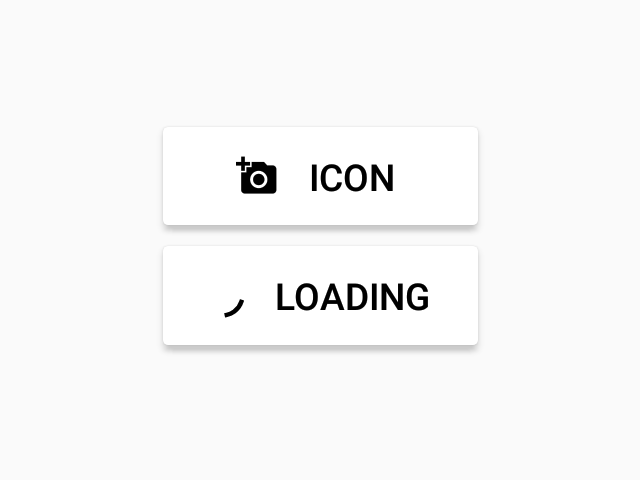
Usage
import * as React from 'react';
import { Button } from 'react-native-paper';
const MyComponent = () => (
<Button raised onPress={() => console.log('Pressed')}>
Press me
</Button>
);
Props
disabled
Type:
boolean
Whether the button is disabled. A disabled button is greyed out and onPress
is not called on touch.
raised
Type:
boolean
Add elevation to button, as opposed to default flat appearance. Typically used on a flat surface.
style
Type:
any