TextInput
TextInputs allow users to input text.
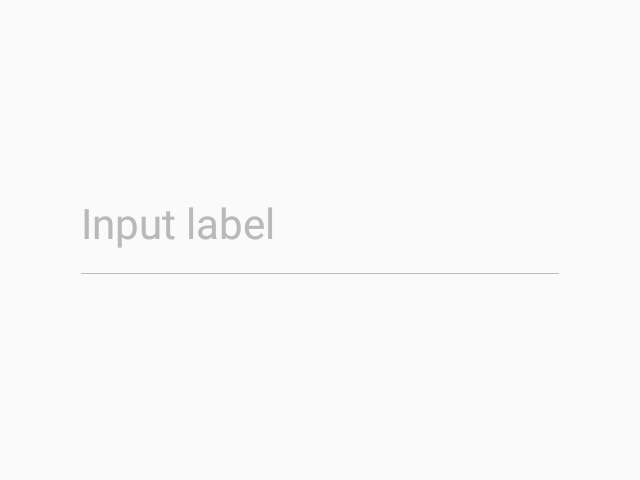
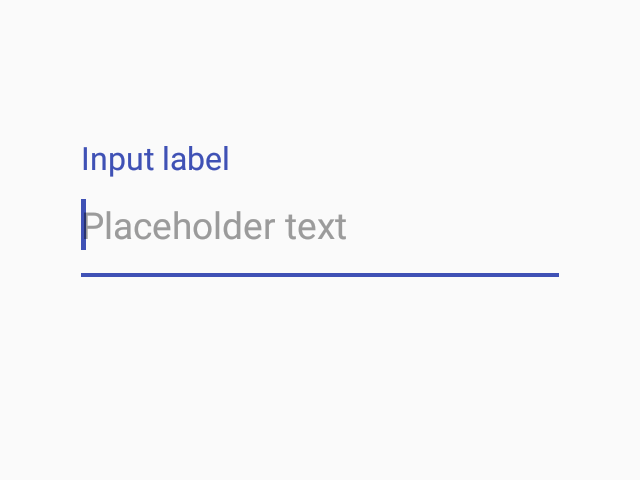
Usage
import * as React from 'react';
import { TextInput } from 'react-native-paper';
class MyComponent extends React.Component {
state = {
text: ''
};
render(){
return (
<TextInput
label='Email'
value={this.state.text}
onChangeText={text => this.setState({ text })}
/>
);
}
}
Props
disabled
Type:
boolean
Default value:
false
If true, user won't be able to interact with the component.
onChangeText
Type:
Function
Callback that is called when the text input's text changes. Changed text is passed as an argument to the callback handler.
selectionColor
Type:
string
Color for the text selection background. Defaults to the theme's primary color.
style
Type:
any
...TextInput props
Methods
isFocused
Returns true
if the input is currently focused, false
otherwise.
clear
Removes all text from the TextInput.
focus
Focuses the input.
blur
Removes focus from the input.