Searchbar
Searchbar is a simple input box where users can type search queries.
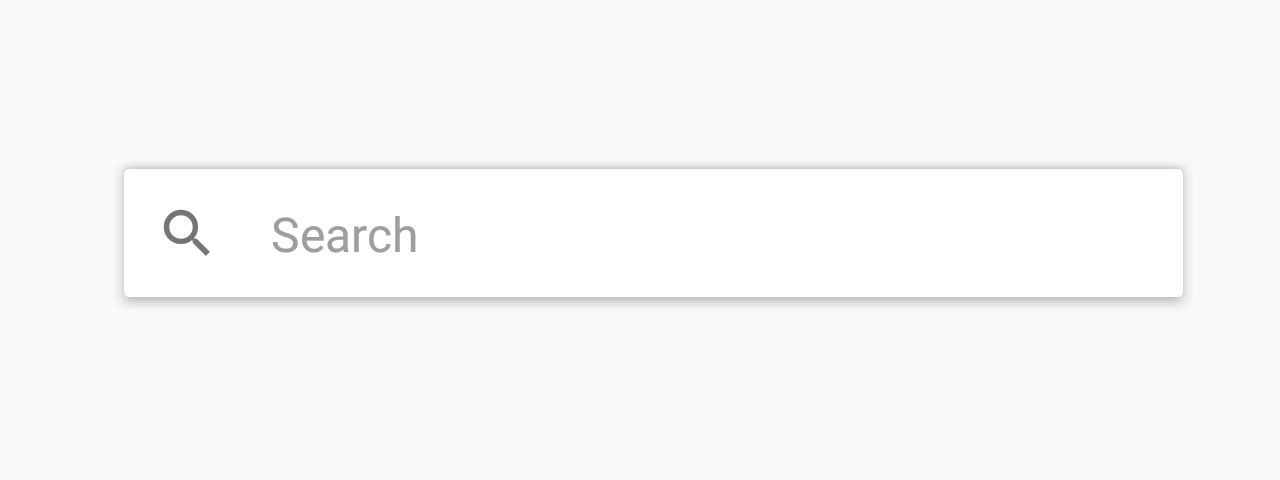
Usage
import React from 'react';
import { Searchbar } from 'react-native-paper';
export default class MyComponent extends React.Component {
state = {
firstQuery: '',
};
render() {
const { firstQuery } = this.state;
return (
<Searchbar
placeholder="Search"
onChangeText={query => { this.setState({ firstQuery: query }); }}
value={firstQuery}
/>
);
}
}
Props
onChangeText
Type:
(query: string) => void
Callback that is called when the text input's text changes.
style
Type:
any
Methods
isFocused
Returns true
if the input is currently focused, false
otherwise.
clear
Removes all text from the TextInput.
focus
Focuses the input.
blur
Removes focus from the input.