Switch
Switch is a visual toggle between two mutually exclusive states — on and off.
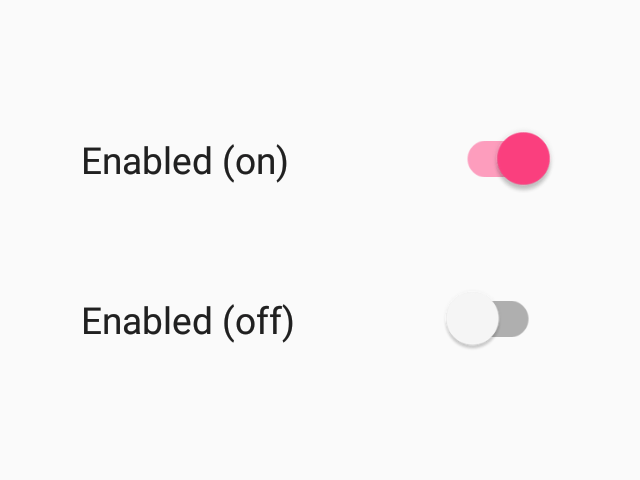
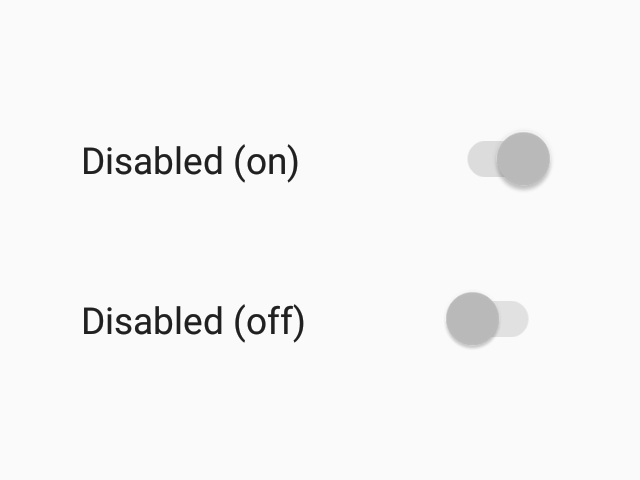
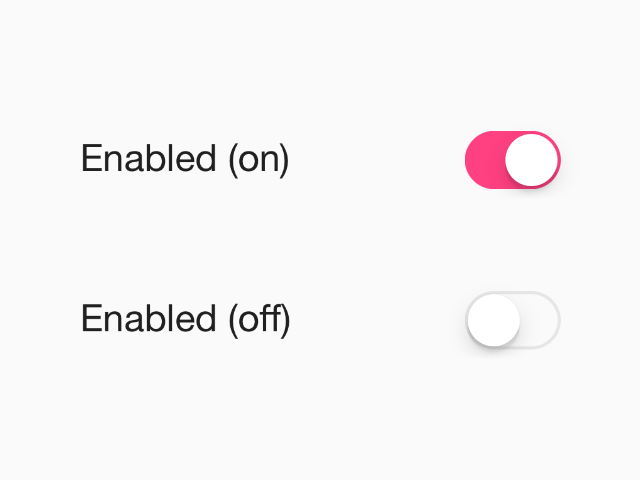
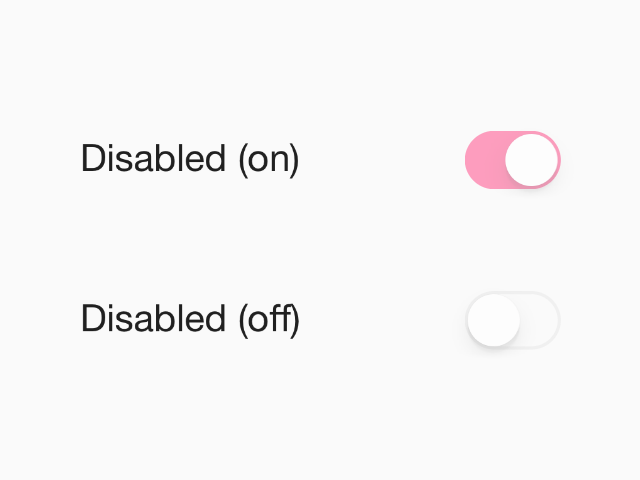
Usage
import * as React from 'react';
import { Switch } from 'react-native-paper';
export default class MyComponent extends React.Component {
state = {
isSwitchOn: false,
};
render() {
const { isSwitchOn } = this.state;
return (
<Switch
value={isSwitchOn}
onValueChange={() =>
{ this.setState({ isSwitchOn: !isSwitchOn }); }
}
/>
);
}
}
Props
style
Type:
any