Card
A card is a sheet of material that serves as an entry point to more detailed information.
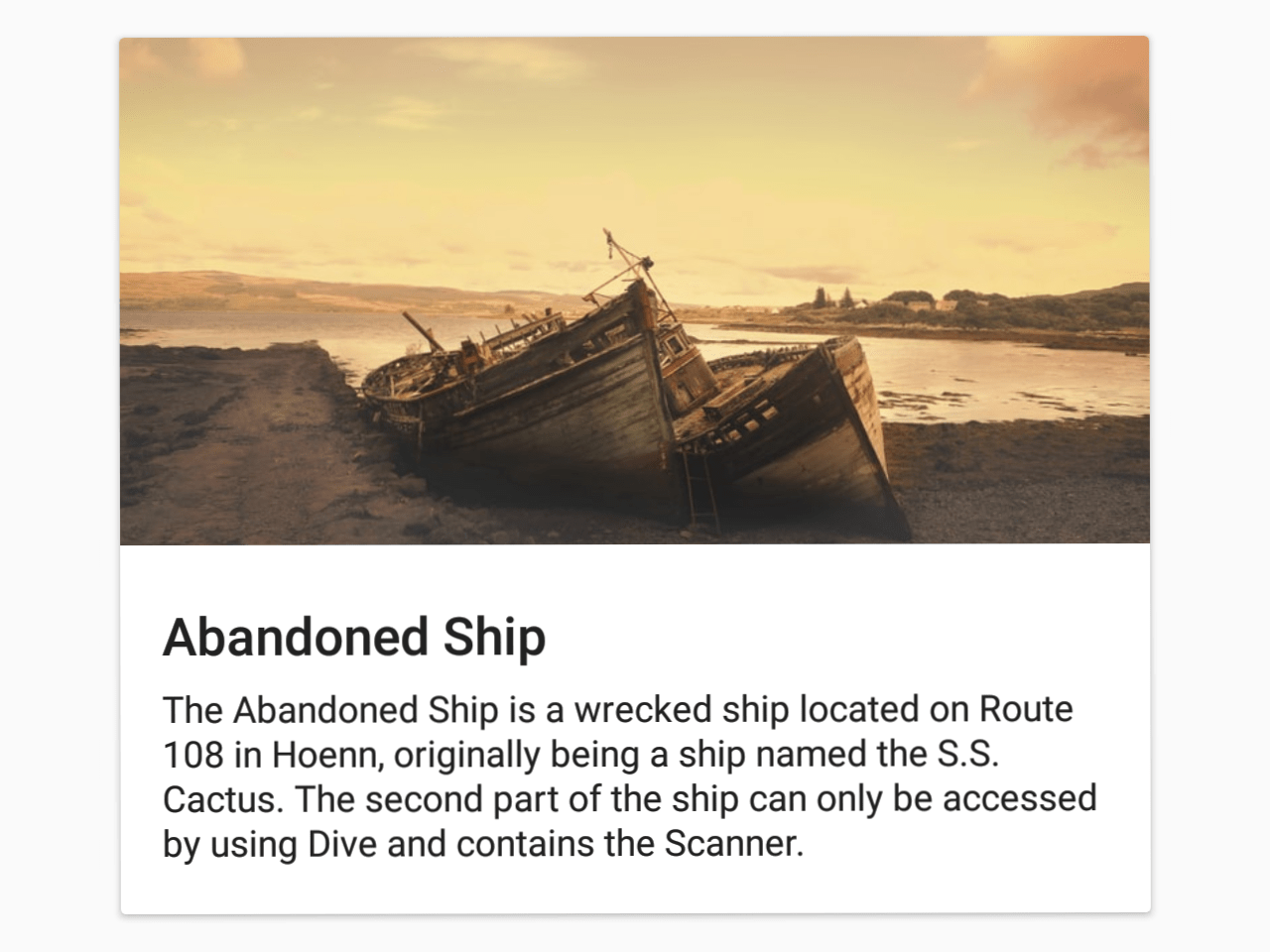
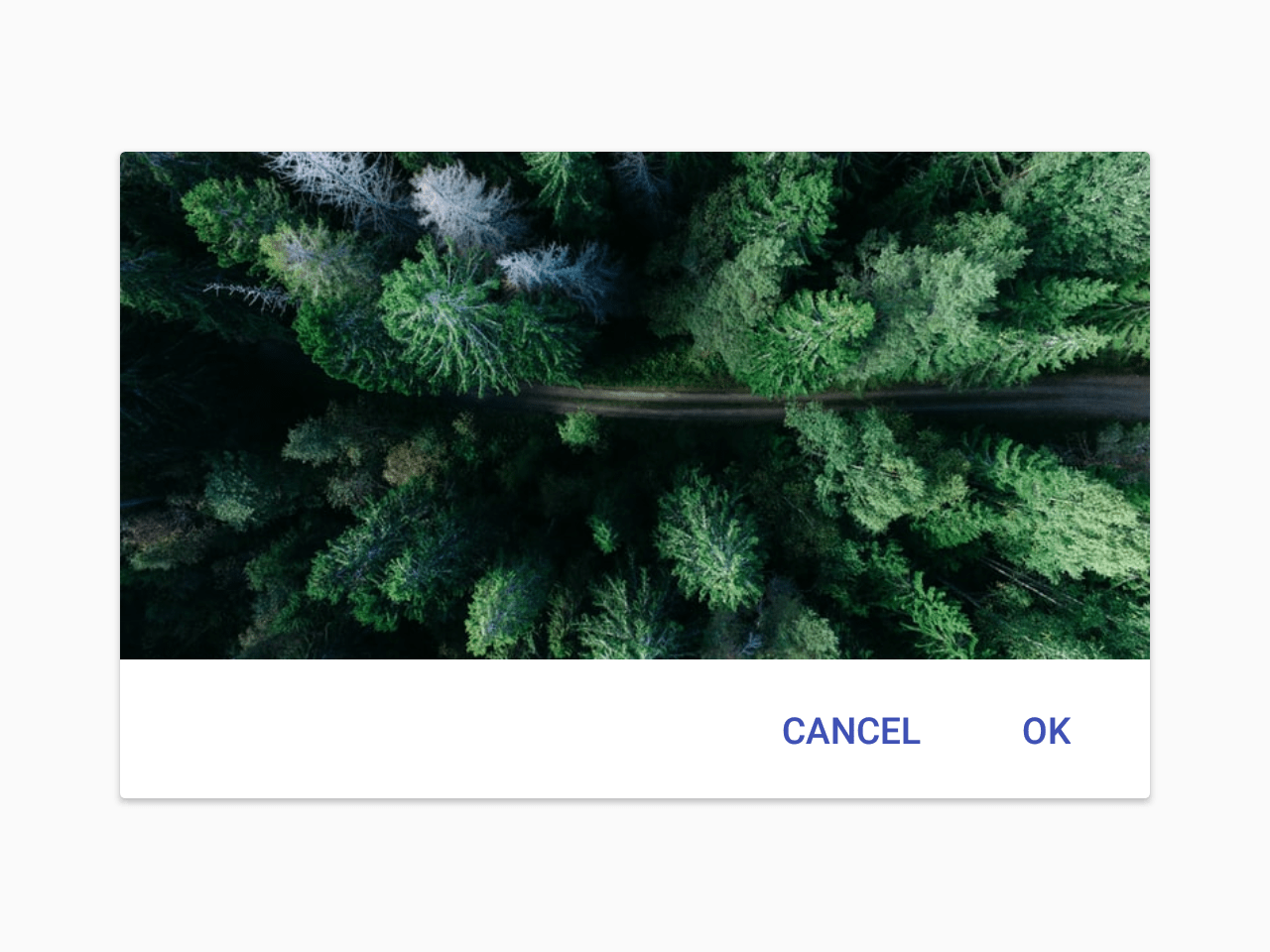
Usage
import * as React from 'react';
import {
Button,
Card,
CardActions,
CardContent,
CardCover,
Title,
Paragraph
} from 'react-native-paper';
const MyComponent = () => (
<Card>
<CardContent>
<Title>Card title</Title>
<Paragraph>Card content</Paragraph>
</CardContent>
<CardCover source={{ uri: 'https://picsum.photos/700' }} />
<CardActions>
<Button>Cancel</Button>
<Button>Ok</Button>
</CardActions>
</Card>
);
Props
style
Type:
any