RadioButton
Radio buttons allow the selection a single option from a set.
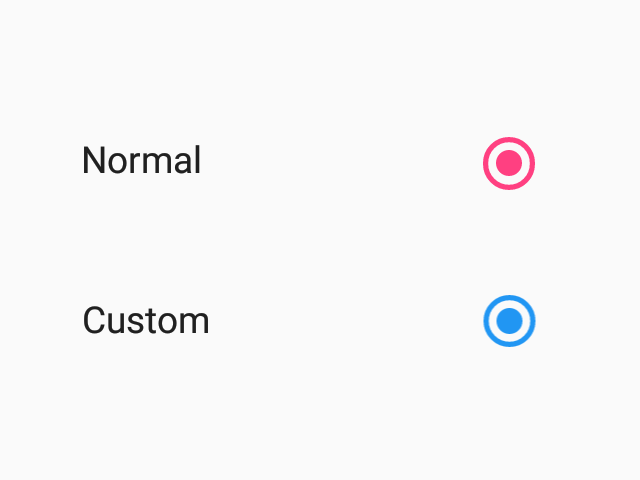
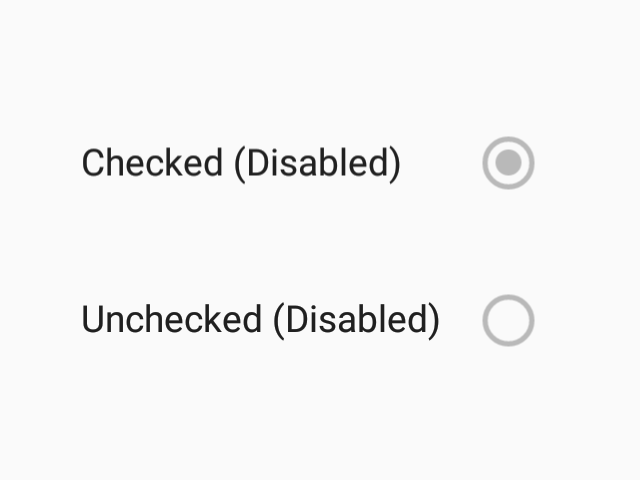
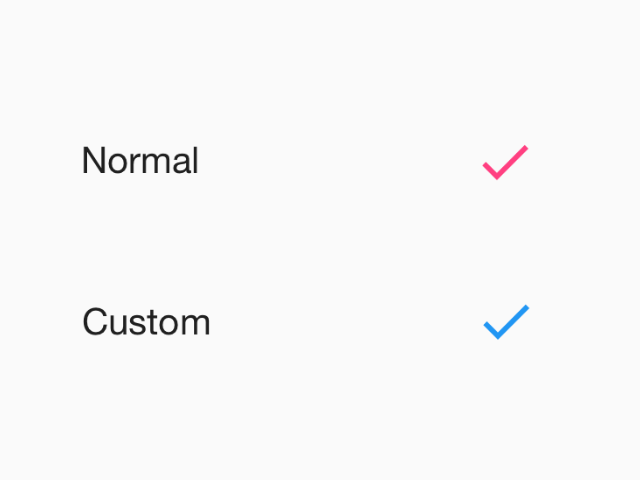
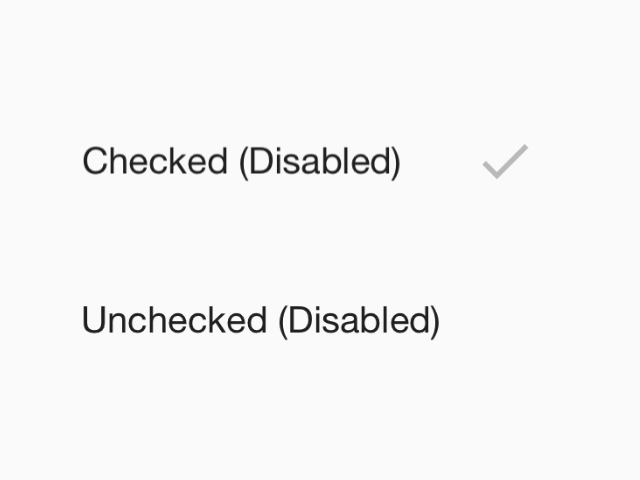
Usage
import * as React from 'react';
import { View } from 'react-native';
import { RadioButton } from 'react-native-paper';
export default class MyComponent extends React.Component {
state = {
checked: 'first',
};
render() {
const { checked } = this.state;
return (
<View>
<RadioButton
value="first"
checked={checked === 'first'}
onPress={() => { this.setState({ checked: 'firstOption' }); }}
/>
<RadioButton
value="second"
checked={checked === 'second'}
onPress={() => { this.setState({ checked: 'secondOption' }); }}
/>
</View>
);
}
}