DataTable
Data tables allow displaying sets of data.
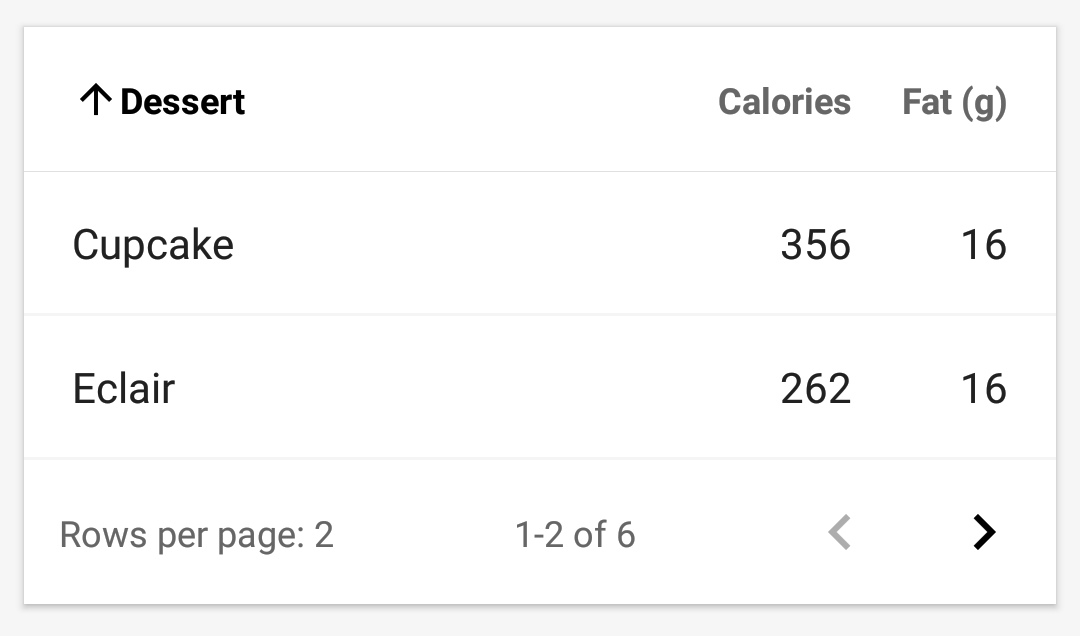
Usage
import * as React from 'react';
import { DataTable } from 'react-native-paper';
const MyComponent = () => (
<DataTable>
<DataTable.Header>
<DataTable.Title>Dessert</DataTable.Title>
<DataTable.Title numeric>Calories</DataTable.Title>
<DataTable.Title numeric>Fat</DataTable.Title>
</DataTable.Header>
<DataTable.Row>
<DataTable.Cell>Frozen yogurt</DataTable.Cell>
<DataTable.Cell numeric>159</DataTable.Cell>
<DataTable.Cell numeric>6.0</DataTable.Cell>
</DataTable.Row>
<DataTable.Row>
<DataTable.Cell>Ice cream sandwich</DataTable.Cell>
<DataTable.Cell numeric>237</DataTable.Cell>
<DataTable.Cell numeric>8.0</DataTable.Cell>
</DataTable.Row>
<DataTable.Pagination
page={1}
numberOfPages={3}
onPageChange={page => {
console.log(page);
}}
label="1-2 of 6"
/>
</DataTable>
);
export default MyComponent;
Props
style
Type:
StyleProp<ViewStyle>
Static properties
These properties can be accessed on DataTable
by using the dot notation, e.g. DataTable.Header
.
Header
Type:
static
Title
Type:
static
Row
Type:
static
Cell
Type:
static
Pagination
Type:
static