Snackbar
Snackbars provide brief feedback about an operation through a message at the bottom of the screen. Snackbar by default use onSurface color from theme
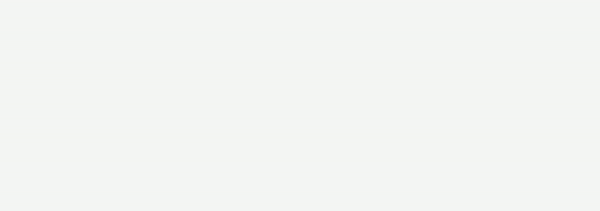
Usage
import * as React from 'react';
import { View, StyleSheet } from 'react-native';
import { Button, Snackbar } from 'react-native-paper';
const MyComponent = () => {
const [visible, setVisible] = React.useState(false);
const onToggleSnackBar = () => setVisible(!visible);
const onDismissSnackBar = () => setVisible(false);
return (
<View style={styles.container}>
<Button onPress={onToggleSnackBar}>{visible ? 'Hide' : 'Show'}</Button>
<Snackbar
visible={visible}
onDismiss={onDismissSnackBar}
action={{
label: 'Undo',
onPress: () => {
// Do something
},
}}>
Hey there! I'm a Snackbar.
</Snackbar>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'space-between',
},
});
export default MyComponent;
Props
action
Type:
{
label: string;
accessibilityLabel?: string;
onPress: () => void;
}
Label and press callback for the action button. It should contain the following properties:
label
- Label of the action buttononPress
- Callback that is called when action button is pressed.
onDismiss
(required)Type:
() => void
Callback called when Snackbar is dismissed. The visible
prop needs to be updated when this is called.
style
Type:
StyleProp<ViewStyle>
ref
Type:
React.RefObject<View>
theme
Type:
Theme
Static properties
These properties can be accessed on Snackbar
by using the dot notation, e.g. Snackbar.DURATION_SHORT
.
DURATION_SHORT
Show the Snackbar for a short duration.
DURATION_MEDIUM
Show the Snackbar for a medium duration.
DURATION_LONG
Show the Snackbar for a long duration.