ToggleButton
Toggle buttons can be used to group related options. To emphasize groups of related toggle buttons, a group should share a common container.
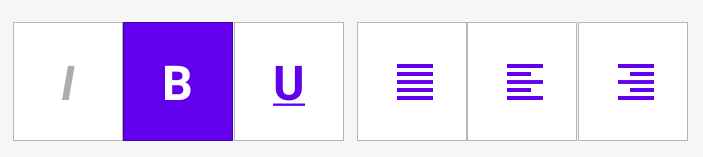
Usage
import * as React from 'react';
import { ToggleButton } from 'react-native-paper';
const ToggleButtonExample = () => {
const [status, setStatus] = React.useState('checked');
const onButtonToggle = value => {
setStatus(status === 'checked' ? 'unchecked' : 'checked');
};
return (
<ToggleButton
icon="bluetooth"
value="bluetooth"
status={status}
onPress={onButtonToggle}
/>
);
};
export default ToggleButtonExample;
Props
accessibilityLabel
Type:
string
Accessibility label for the ToggleButton
. This is read by the screen reader when the user taps the button.
style
Type:
StyleProp<ViewStyle>
theme
Type:
Theme
Static properties
These properties can be accessed on ToggleButton
by using the dot notation, e.g. ToggleButton.Group
.
Group
Type:
static
Row
Type:
static