Banner
Banner displays a prominent message and related actions.
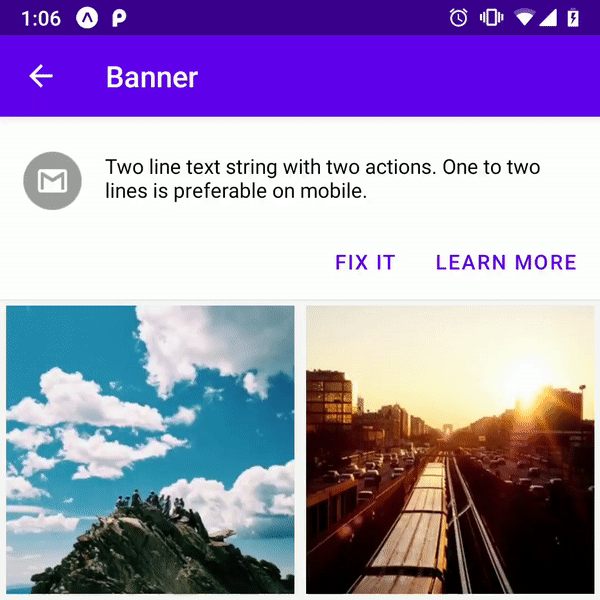
Usage
import * as React from 'react';
import { Image } from 'react-native';
import { Banner } from 'react-native-paper';
export default class MyComponent extends React.Component {
state = {
visible: true,
};
render() {
return (
<Banner
visible={this.state.visible}
actions={[
{
label: 'Fix it',
onPress: () => this.setState({ visible: false }),
},
{
label: 'Learn more',
onPress: () => this.setState({ visible: false }),
},
]}
image={({ size }) =>
<Image
source={{ uri: 'https://avatars3.githubusercontent.com/u/17571969?s=400&v=4' }}
style={{
width: size,
height: size,
}}
/>
}
>
There was a problem processing a transaction on your credit card.
</Banner>
);
}
}
Props
image
Type:
(props: { size: number }) => React.Node
Callback that returns an image to display inside banner.
actions
(required)Type:
Array<{
label: string,
onPress: () => mixed,
}>
Action items to shown in the banner. An action item should contain the following properties:
label
: label of the action button (required)onPress
: callback that is called when button is pressed (required)
To customize button you can pass other props that button component takes.
style
Type:
any
theme
Type:
Theme