Snackbar
Snackbars provide brief feedback about an operation through a message at the bottom of the screen.
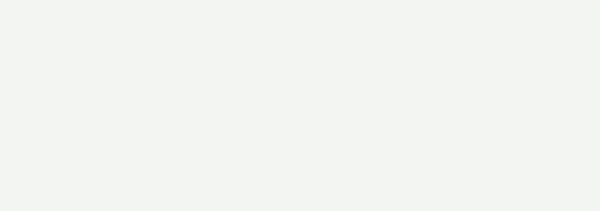
Usage
import * as React from 'react';
import { StyleSheet } from 'react-native';
import { Snackbar } from 'react-native-paper';
export default class MyComponent extends React.Component {
state = {
visible: false,
};
render() {
const { visible } = this.state;
return (
<View style={styles.container}>
<Button
onPress={() => this.setState(state => ({ visible: !state.visible }))}
>
{this.state.visible ? 'Hide' : 'Show'}
</Button>
<Snackbar
visible={this.state.visible}
onDismiss={() => this.setState({ visible: false })}
action={{
label: 'Undo',
onPress: () => {
// Do something
},
}}
>
Hey there! I'm a Snackbar.
</Snackbar>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'space-between',
},
});
Props
action
Type:
{
label: string,
accessibilityLabel?: string,
onPress: () => mixed,
}
Label and press callback for the action button. It should contain the following properties:
label
- Label of the action buttononPress
- Callback that is called when action button is pressed.
onDismiss
(required)Type:
() => mixed
Callback called when Snackbar is dismissed. The visible
prop needs to be updated when this is called.
style
Type:
any
theme
Type:
Theme
Static properties
These properties can be accessed on Snackbar
by using the dot notation, e.g. Snackbar.DURATION_SHORT
.
DURATION_SHORT
Show the Snackbar for a short duration.
DURATION_MEDIUM
Show the Snackbar for a medium duration.
DURATION_LONG
Show the Snackbar for a long duration.