BottomNavigation.Bar
A navigation bar which can easily be integrated with React Navigation's Bottom Tabs Navigator.
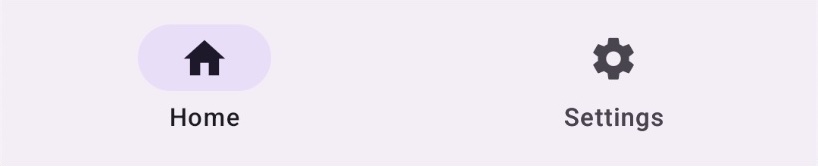
Usage
without React Navigation
import React from 'react';
import { useState } from 'react';
import { View } from 'react-native';
import { BottomNavigation, Text, Provider } from 'react-native-paper';
import MaterialCommunityIcons from '@expo/vector-icons/MaterialCommunityIcons';
function HomeScreen() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Home!</Text>
</View>
);
}
function SettingsScreen() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Settings!</Text>
</View>
);
}
export default function MyComponent() {
const [index, setIndex] = useState(0);
const routes = [
{ key: 'home', title: 'Home', icon: 'home' },
{ key: 'settings', title: 'Settings', icon: 'cog' },
];
const renderScene = ({ route }) => {
switch (route.key) {
case 'home':
return <HomeScreen />;
case 'settings':
return <SettingsScreen />;
default:
return null;
}
};
return (
<Provider>
{renderScene({ route: routes[index] })}
<BottomNavigation.Bar
navigationState={{ index, routes }}
onTabPress={({ route }) => {
const newIndex = routes.findIndex((r) => r.key === route.key);
if (newIndex !== -1) {
setIndex(newIndex);
}
}}
renderIcon={({ route, color }) => (
<Icon name={route.icon} size={24} color={color} />
)}
getLabelText={({ route }) => route.title}
/>
</Provider>
);
}
with React Navigation
- static
- dynamic
import { Text, View } from 'react-native';
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
import { Provider, BottomNavigation } from 'react-native-paper';
import MaterialCommunityIcons from '@expo/vector-icons/MaterialCommunityIcons';
import {
CommonActions,
createStaticNavigation,
} from '@react-navigation/native';
function HomeScreen() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Home!</Text>
</View>
);
}
function SettingsScreen() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Settings!</Text>
</View>
);
}
const MyTabs = createBottomTabNavigator({
screenOptions: {
animation: 'shift',
},
tabBar: ({ navigation, state, descriptors, insets }) => (
<BottomNavigation.Bar
navigationState={state}
safeAreaInsets={insets}
onTabPress={({ route, preventDefault }) => {
const event = navigation.emit({
type: 'tabPress',
target: route.key,
canPreventDefault: true,
});
if (event.defaultPrevented) {
preventDefault();
} else {
navigation.dispatch({
...CommonActions.navigate(route.name, route.params),
target: state.key,
});
}
}}
renderIcon={({ route, focused, color }) =>
descriptors[route.key].options.tabBarIcon?.({
focused,
color,
size: 24,
}) || null
}
getLabelText={({ route }) => {
const { options } = descriptors[route.key];
const label =
typeof options.tabBarLabel === 'string'
? options.tabBarLabel
: typeof options.title === 'string'
? options.title
: route.name;
return label;
}}
/>
),
screens: {
Home: {
screen: HomeScreen,
options: {
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="home" color={color} size={26} />
),
},
},
Settings: {
screen: SettingsScreen,
options: {
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="cog" color={color} size={26} />
),
},
},
},
});
const Navigation = createStaticNavigation(MyTabs);
export default function App() {
return (
<Provider>
<Navigation>
<MyTabs />
</Navigation>
</Provider>
);
}
import { Text, View } from 'react-native';
import { NavigationContainer, CommonActions } from '@react-navigation/native';
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
import { Provider, BottomNavigation } from 'react-native-paper';
import MaterialCommunityIcons from '@expo/vector-icons/MaterialCommunityIcons';
function HomeScreen() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Home!</Text>
</View>
);
}
function SettingsScreen() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Settings!</Text>
</View>
);
}
const Tab = createBottomTabNavigator();
export default function App() {
return (
<Provider>
<NavigationContainer>
<Tab.Navigator
screenOptions={{
animation: 'shift',
}}
tabBar={({ navigation, state, descriptors, insets }) => (
<BottomNavigation.Bar
navigationState={state}
safeAreaInsets={insets}
onTabPress={({ route, preventDefault }) => {
const event = navigation.emit({
type: 'tabPress',
target: route.key,
canPreventDefault: true,
});
if (event.defaultPrevented) {
preventDefault();
} else {
navigation.dispatch({
...CommonActions.navigate(route.name, route.params),
target: state.key,
});
}
}}
renderIcon={({ route, focused, color }) =>
descriptors[route.key].options.tabBarIcon?.({
focused,
color,
size: 24,
}) || null
}
getLabelText={({ route }) => {
const { options } = descriptors[route.key];
const label =
typeof options.tabBarLabel === 'string'
? options.tabBarLabel
: typeof options.title === 'string'
? options.title
: route.name;
return label;
}}
/>
)}>
<Tab.Screen
name="Home"
component={HomeScreen}
options={{
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="home" color={color} size={26} />
),
}}
/>
<Tab.Screen
name="Settings"
component={SettingsScreen}
options={{
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="cog" color={color} size={26} />
),
}}
/>
</Tab.Navigator>
</NavigationContainer>
</Provider>
);
}
Props
shifting
Type: boolean
Whether the shifting style is used, the active tab icon shifts up to show the label and the inactive tabs won't have a label.
By default, this is false
with theme version 3 and true
when you have more than 3 tabs.
Pass shifting={false}
to explicitly disable this animation, or shifting={true}
to always use this animation.
Note that you need at least 2 tabs be able to run this animation.
labeled
Type: boolean
Default value: true
Whether to show labels in tabs. When false
, only icons will be displayed.
compact
Type: boolean
Whether tabs should be spread across the entire width.
navigationState (required)
Type: {
index: number;
routes: Route[];
}
State for the bottom navigation. The state should contain the following properties:
index
: a number representing the index of the active route in theroutes
arrayroutes
: an array containing a list of route objects used for rendering the tabs
Each route object should contain the following properties:
key
: a unique key to identify the route (required)title
: title of the route to use as the tab labelfocusedIcon
: icon to use as the focused tab icon, can be a string, an image source or a react component Renamed from 'icon' to 'focusedIcon' in v5.xunfocusedIcon
: icon to use as the unfocused tab icon, can be a string, an image source or a react component Available in v5.x with theme version 3color
: color to use as background color for shifting bottom navigation In v5.x works only with theme version 2.badge
: badge to show on the tab icon, can betrue
to show a dot,string
ornumber
to show text.accessibilityLabel
: accessibility label for the tab buttontestID
: test id for the tab button
Example:
{
index: 1,
routes: [
{ key: 'music', title: 'Favorites', focusedIcon: 'heart', unfocusedIcon: 'heart-outline'},
{ key: 'albums', title: 'Albums', focusedIcon: 'album' },
{ key: 'recents', title: 'Recents', focusedIcon: 'history' },
{ key: 'notifications', title: 'Notifications', focusedIcon: 'bell', unfocusedIcon: 'bell-outline' },
]
}
BottomNavigation.Bar
is a controlled component, which means the index
needs to be updated via the onTabPress
callback.
renderIcon
Type: (props: {
route: Route;
focused: boolean;
color: string;
}) => React.ReactNode
Callback which returns a React Element to be used as tab icon.
renderLabel
Type: (props: {
route: Route;
focused: boolean;
color: string;
}) => React.ReactNode
Callback which React Element to be used as tab label.
renderTouchable
Type: (props: TouchableProps<Route>) => React.ReactNode
Default value: ({ key, ...props }: TouchableProps<Route>) => (
<Touchable key={key} {...props} />
)
Callback which returns a React element to be used as the touchable for the tab item.
Renders a TouchableRipple
on Android and Pressable
on iOS.
getAccessibilityLabel
Type: (props: { route: Route }) => string | undefined
Default value: ({ route }: { route: Route }) =>
route.accessibilityLabel
Get accessibility label for the tab button. This is read by the screen reader when the user taps the tab.
Uses route.accessibilityLabel
by default.
getBadge
Type: (props: { route: Route }) => boolean | number | string | undefined
Default value: ({ route }: { route: Route }) => route.badge
Get badge for the tab, uses route.badge
by default.
getColor
Type: (props: { route: Route }) => string | undefined
Default value: ({ route }: { route: Route }) => route.color
Get color for the tab, uses route.color
by default.
getLabelText
Type: (props: { route: Route }) => string | undefined
Default value: ({ route }: { route: Route }) => route.title
Get label text for the tab, uses route.title
by default. Use renderLabel
to replace label component.
getTestID
Type: (props: { route: Route }) => string | undefined
Default value: ({ route }: { route: Route }) => route.testID
Get the id to locate this tab button in tests, uses route.testID
by default.
onTabPress (required)
Type: (props: { route: Route } & TabPressEvent) => void
Function to execute on tab press. It receives the route for the pressed tab. Use this to update the navigation state.
onTabLongPress
Type: (props: { route: Route } & TabPressEvent) => void
Function to execute on tab long press. It receives the route for the pressed tab
activeColor
Type: string
Custom color for icon and label in the active tab.
inactiveColor
Type: string
Custom color for icon and label in the inactive tab.
animationEasing
Type: EasingFunction | undefined
The scene animation Easing.
keyboardHidesNavigationBar
Type: boolean
Default value: Platform.OS === 'android'
Whether the bottom navigation bar is hidden when keyboard is shown.
On Android, this works best when windowSoftInputMode
is set to adjustResize
.
safeAreaInsets
Type: {
top?: number;
right?: number;
bottom?: number;
left?: number;
}
Safe area insets for the tab bar. This can be used to avoid elements like the navigation bar on Android and bottom safe area on iOS. The bottom insets for iOS is added by default. You can override the behavior with this option.
labelMaxFontSizeMultiplier
Type: number
Default value: 1
Specifies the largest possible scale a label font can reach.
style
Type: Animated.WithAnimatedValue<StyleProp<ViewStyle>>
activeIndicatorStyle
Type: StyleProp<ViewStyle>
theme
Type: ThemeProp
testID
Type: string
Default value: 'bottom-navigation-bar'
TestID used for testing purposes