ToggleButton
Toggle buttons can be used to group related options. To emphasize groups of related toggle buttons, a group should share a common container.
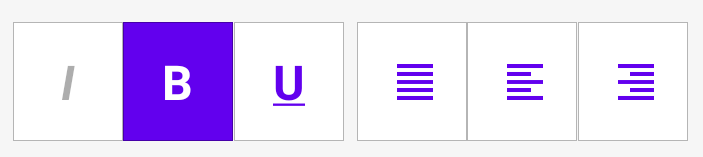
Usage
import * as React from 'react';
import { ToggleButton } from 'react-native-paper';
const ToggleButtonExample = () => {
const [status, setStatus] = React.useState('checked');
const onButtonToggle = value => {
setStatus(status === 'checked' ? 'unchecked' : 'checked');
};
return (
<ToggleButton
icon="bluetooth"
value="bluetooth"
status={status}
onPress={onButtonToggle}
/>
);
};
export default ToggleButtonExample;
Props
icon (required)
Type: IconSource
Icon to display for the ToggleButton
.
size
Type: number
Size of the icon.
iconColor
Type: string
Custom text color for button.
rippleColor
Type: ColorValue
Color of the ripple effect.
disabled
Type: boolean
Whether the button is disabled.
accessibilityLabel
Type: string
Accessibility label for the ToggleButton
. This is read by the screen reader when the user taps the button.
onPress
Type: (value?: GestureResponderEvent | string) => void
Function to execute on press.
value
Type: string
Value of button.
status
Type: 'checked' | 'unchecked'
Status of button.
style
Type: Animated.WithAnimatedValue<StyleProp<ViewStyle>>
theme
Type: ThemeProp
ref
Type: React.RefObject<View>
testID
Type: string
testID to be used on tests.