Drawer.Item
A component used to show an action item with an icon and a label in a navigation drawer.
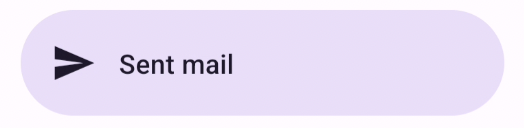
Usage
import * as React from 'react';
import { Drawer } from 'react-native-paper';
const MyComponent = () => (
<Drawer.Item
style={{ backgroundColor: '#64ffda' }}
icon="star"
label="First Item"
/>
);
export default MyComponent;
Props
label (required)
Type: string
The label text of the item.
icon
Type: IconSource
Icon to display for the DrawerItem
.
active
Type: boolean
Whether to highlight the drawer item as active.
disabled
Type: boolean
Whether the item is disabled.
onPress
Type: (e: GestureResponderEvent) => void
Function to execute on press.
background
Type: PressableAndroidRippleConfig
Type of background drawabale to display the feedback (Android). https://reactnative.dev/docs/pressable#rippleconfig
accessibilityLabel
Type: string
Accessibility label for the button. This is read by the screen reader when the user taps the button.
right
Type: (props: { color: string }) => React.ReactNode
Callback which returns a React element to display on the right side. For instance a Badge.
labelMaxFontSizeMultiplier
Type: number
Specifies the largest possible scale a label font can reach.
rippleColor
Type: ColorValue
Color of the ripple effect.
style
Type: StyleProp<ViewStyle>
theme
Type: ThemeProp
Theme colors
mode | backgroundColor | iconColor/textColor |
---|---|---|
active | theme.colors.secondaryContainer | theme.colors.onSecondaryContainer |
inactive | theme.colors.onSurfaceVariant |
If a dedicated prop for a specific color is not available or the style
prop does not allow color modification, you can customize it using the theme
prop. It allows to override any color, within the component, based on the table above.
Example of overriding primary
color:
<Drawer.Item theme={{ colors: { primary: 'green' } }} />