FAB.Group
A component to display a stack of FABs with related actions in a speed dial.
To render the group above other components, you'll need to wrap it with the Portal
component.
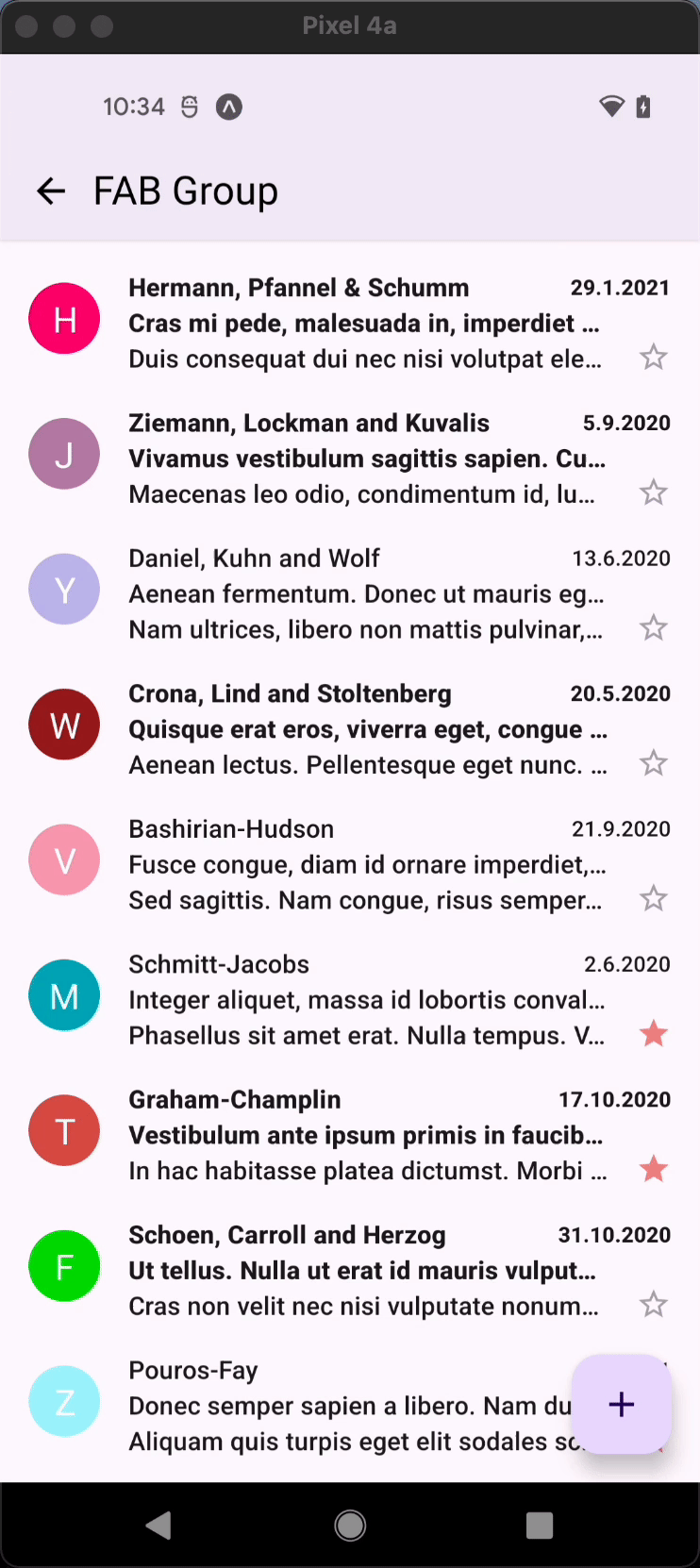
Usage
import * as React from 'react';
import { FAB, Portal, PaperProvider } from 'react-native-paper';
const MyComponent = () => {
const [state, setState] = React.useState({ open: false });
const onStateChange = ({ open }) => setState({ open });
const { open } = state;
return (
<PaperProvider>
<Portal>
<FAB.Group
open={open}
visible
icon={open ? 'calendar-today' : 'plus'}
actions={[
{ icon: 'plus', onPress: () => console.log('Pressed add') },
{
icon: 'star',
label: 'Star',
onPress: () => console.log('Pressed star'),
},
{
icon: 'email',
label: 'Email',
onPress: () => console.log('Pressed email'),
},
{
icon: 'bell',
label: 'Remind',
onPress: () => console.log('Pressed notifications'),
},
]}
onStateChange={onStateChange}
onPress={() => {
if (open) {
// do something if the speed dial is open
}
}}
/>
</Portal>
</PaperProvider>
);
};
export default MyComponent;
Props
actions (required)
Type: Array<{
icon: IconSource;
label?: string;
color?: string;
labelTextColor?: string;
accessibilityLabel?: string;
accessibilityHint?: string;
style?: Animated.WithAnimatedValue<StyleProp<ViewStyle>>;
containerStyle?: Animated.WithAnimatedValue<StyleProp<ViewStyle>>;
wrapperStyle?: StyleProp<ViewStyle>;
labelStyle?: StyleProp<TextStyle>;
labelMaxFontSizeMultiplier?: number;
onPress: (e: GestureResponderEvent) => void;
size?: 'small' | 'medium';
testID?: string;
rippleColor?: ColorValue;
}>
Action items to display in the form of a speed dial. An action item should contain the following properties:
icon
: icon to display (required)label
: optional label textcolor
: custom icon color of the action itemlabelTextColor
: custom label text color of the action itemaccessibilityLabel
: accessibility label for the action, uses label by default if specifiedaccessibilityHint
: accessibility hint for the actionstyle
: pass additional styles for the fab item, for example,backgroundColor
containerStyle
: pass additional styles for the fab item label container, for example,backgroundColor
Available in 5.xwrapperStyle
: pass additional styles for the wrapper of the action item.labelStyle
: pass additional styles for the fab item label, for example,fontSize
labelMaxFontSizeMultiplier
: specifies the largest possible scale a title font can reach.onPress
: callback that is called whenFAB
is pressed (required)onLongPress
: callback that is called whenFAB
is long pressedtoggleStackOnLongPress
: callback that is called whenFAB
is long pressedsize
: size of action item. Defaults tosmall
. Available in v5.xtestID
: testID to be used on testsrippleColor
: color of the ripple effect.
icon (required)
Type: IconSource
Icon to display for the FAB
.
You can toggle it based on whether the speed dial is open to display a different icon.
accessibilityLabel
Type: string
Accessibility label for the FAB. This is read by the screen reader when the user taps the FAB.
color
Type: string
Custom color for the FAB
.
backdropColor
Type: string
Custom backdrop color for opened speed dial background.
rippleColor
Type: ColorValue
Color of the ripple effect.
onPress
Type: (e: GestureResponderEvent) => void
Function to execute on pressing the FAB
.
onLongPress
Type: (e: GestureResponderEvent) => void
Function to execute on long pressing the FAB
.
toggleStackOnLongPress
Type: boolean
Makes actions stack appear on long press instead of on press.
delayLongPress
Type: number
Default value: 200
Changes the delay for long press reaction.
enableLongPressWhenStackOpened
Type: boolean
Default value: false
Allows for onLongPress when stack is opened.
open (required)
Type: boolean
Whether the speed dial is open.
onStateChange (required)
Type: (state: { open: boolean }) => void
Callback which is called on opening and closing the speed dial. The open state needs to be updated when it's called, otherwise the change is dropped.
visible (required)
Type: boolean
Whether FAB
is currently visible.
style
Type: StyleProp<ViewStyle>
Style for the group. You can use it to pass additional styles if you need. For example, you can set an additional padding if you have a tab bar at the bottom.
fabStyle
Type: Animated.WithAnimatedValue<StyleProp<ViewStyle>>
Style for the FAB. It allows to pass the FAB button styles, such as backgroundColor.
variant Available in v5.x with theme version 3
Type: 'primary' | 'secondary' | 'tertiary' | 'surface'
Default value: 'primary'
Color mappings variant for combinations of container and icon colors.
theme
Type: ThemeProp
label
Type: string
Optional label for FAB
.
testID
Type: string
Pass down testID from Group props to FAB.