BottomNavigation
Bottom navigation provides quick navigation between top-level views of an app with a bottom navigation bar. It is primarily designed for use on mobile.
For integration with React Navigation, you can use react-navigation-material-bottom-tabs and consult createMaterialBottomTabNavigator documentation.
By default Bottom navigation uses primary color as a background, in dark theme with adaptive
mode it will use surface colour instead.
See Dark Theme for more information.
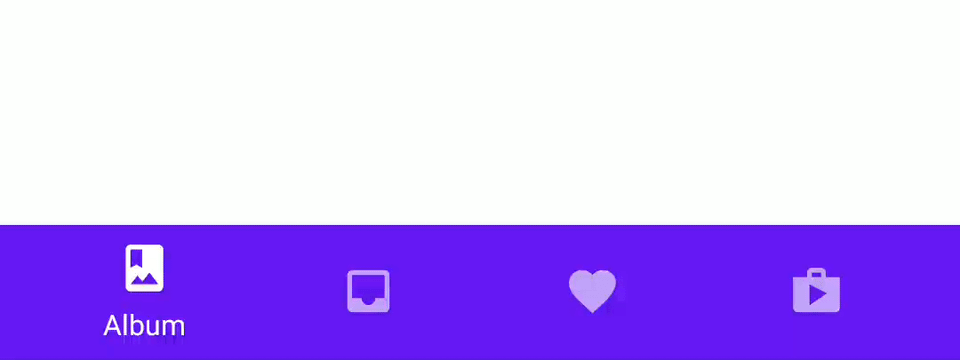
Usage
import * as React from 'react';
import { BottomNavigation, Text } from 'react-native-paper';
const MusicRoute = () => <Text>Music</Text>;
const AlbumsRoute = () => <Text>Albums</Text>;
const RecentsRoute = () => <Text>Recents</Text>;
const MyComponent = () => {
const [index, setIndex] = React.useState(0);
const [routes] = React.useState([
{ key: 'music', title: 'Music', icon: 'queue-music' },
{ key: 'albums', title: 'Albums', icon: 'album' },
{ key: 'recents', title: 'Recents', icon: 'history' },
]);
const renderScene = BottomNavigation.SceneMap({
music: MusicRoute,
albums: AlbumsRoute,
recents: RecentsRoute,
});
return (
<BottomNavigation
navigationState={{ index, routes }}
onIndexChange={setIndex}
renderScene={renderScene}
/>
);
};
export default MyComponent;
Props
shifting
boolean
navigationState.routes.length > 3
Whether the shifting style is used, the active tab icon shifts up to show the label and the inactive tabs won't have a label.
By default, this is true
when you have more than 3 tabs.
Pass shifting={false}
to explicitly disable this animation, or shifting={true}
to always use this animation.
labeled
boolean
true
Whether to show labels in tabs. When false
, only icons will be displayed.
navigationState
(required){
index: number;
routes: Route[];
}
State for the bottom navigation. The state should contain the following properties:
index
: a number representing the index of the active route in theroutes
arrayroutes
: an array containing a list of route objects used for rendering the tabs
Each route object should contain the following properties:
key
: a unique key to identify the route (required)title
: title of the route to use as the tab labelicon
: icon to use as the tab icon, can be a string, an image source or a react componentcolor
: color to use as background color for shifting bottom navigationbadge
: badge to show on the tab icon, can betrue
to show a dot,string
ornumber
to show text.accessibilityLabel
: accessibility label for the tab buttontestID
: test id for the tab button
Example:
{
index: 1,
routes: [
{ key: 'music', title: 'Music', icon: 'queue-music', color: '#3F51B5' },
{ key: 'albums', title: 'Albums', icon: 'album', color: '#009688' },
{ key: 'recents', title: 'Recents', icon: 'history', color: '#795548' },
{ key: 'purchased', title: 'Purchased', icon: 'shopping-cart', color: '#607D8B' },
]
}
BottomNavigation
is a controlled component, which means the index
needs to be updated via the onIndexChange
callback.
onIndexChange
(required)(index: number) => void
Callback which is called on tab change, receives the index of the new tab as argument. The navigation state needs to be updated when it's called, otherwise the change is dropped.
renderScene
(required)(props: {
route: Route;
jumpTo: (key: string) => void;
}) => React.ReactNode | null
Callback which returns a react element to render as the page for the tab. Receives an object containing the route as the argument:
renderScene = ({ route, jumpTo }) => {
switch (route.key) {
case 'music':
return <MusicRoute jumpTo={jumpTo} />;
case 'albums':
return <AlbumsRoute jumpTo={jumpTo} />;
}
}
Pages are lazily rendered, which means that a page will be rendered the first time you navigate to it. After initial render, all the pages stay rendered to preserve their state.
You need to make sure that your individual routes implement a shouldComponentUpdate
to improve the performance.
To make it easier to specify the components, you can use the SceneMap
helper:
renderScene = BottomNavigation.SceneMap({
music: MusicRoute,
albums: AlbumsRoute,
});
Specifying the components this way is easier and takes care of implementing a shouldComponentUpdate
method.
Each component will receive the current route and a jumpTo
method as it's props.
The jumpTo
method can be used to navigate to other tabs programmatically:
this.props.jumpTo('albums')
renderIcon
(props: {
route: Route;
focused: boolean;
color: string;
}) => React.ReactNode
Callback which returns a React Element to be used as tab icon.
renderLabel
(props: {
route: Route;
focused: boolean;
color: string;
}) => React.ReactNode
Callback which React Element to be used as tab label.
renderTouchable
(props: TouchableProps) => React.ReactNode
(props: TouchableProps) => <Touchable {...props} />
Callback which returns a React element to be used as the touchable for the tab item.
Renders a TouchableRipple
on Android and TouchableWithoutFeedback
with View
on iOS.
getLabelText
(props: { route: Route }) => string | undefined
({ route }: { route: Route }) => route.title
Get label text for the tab, uses route.title
by default. Use renderLabel
to replace label component.
getAccessibilityLabel
(props: { route: Route }) => string | undefined
({ route }: { route: Route }) =>
route.accessibilityLabel
Get accessibility label for the tab button. This is read by the screen reader when the user taps the tab.
Uses route.accessibilityLabel
by default.
getTestID
(props: { route: Route }) => string | undefined
({ route }: { route: Route }) => route.testID
Get the id to locate this tab button in tests, uses route.testID
by default.
getBadge
(props: { route: Route }) => boolean | number | string | undefined
({ route }: { route: Route }) => route.badge
Get badge for the tab, uses route.badge
by default.
getColor
(props: { route: Route }) => string | undefined
({ route }: { route: Route }) => route.color
Get color for the tab, uses route.color
by default.
onTabPress
(props: { route: Route } & TabPressEvent) => void
Function to execute on tab press. It receives the route for the pressed tab, useful for things like scroll to top.
sceneAnimationEnabled
boolean
false
Whether animation is enabled for scenes transitions in shifting
mode.
By default, the scenes cross-fade during tab change when shifting
is enabled.
Specify sceneAnimationEnabled
as false
to disable the animation.
keyboardHidesNavigationBar
boolean
true
Whether the bottom navigation bar is hidden when keyboard is shown.
On Android, this works best when windowSoftInputMode
is set to adjustResize
.
safeAreaInsets
{
top?: number;
right?: number;
bottom?: number;
left?: number;
}
Safe area insets for the tab bar. This can be used to avoid elements like the navigation bar on Android and bottom safe area on iOS. The bottom insets for iOS is added by default. You can override the behavior with this option.
barStyle
StyleProp<ViewStyle>
Style for the bottom navigation bar. You can pass a custom background color here:
barStyle={{ backgroundColor: '#694fad' }}
labelMaxFontSizeMultiplier
number
1
Specifies the largest possible scale a label font can reach.
style
StyleProp<ViewStyle>
theme
ReactNativePaper.Theme
Static properties
These properties can be accessed on BottomNavigation
by using the dot notation, e.g. BottomNavigation.SceneMap
.
SceneMap
Function
scenes: {
[key: string]: React.ComponentType<{
route: Route;
jumpTo: (key: string) => void;
}>;
}
Function which takes a map of route keys to components. Pure components are used to minimize re-rendering of the pages. This drastically improves the animation performance.