Dialog
Dialogs inform users about a specific task and may contain critical information, require decisions, or involve multiple tasks.
To render the Dialog
above other components, you'll need to wrap it with the Portal
component.
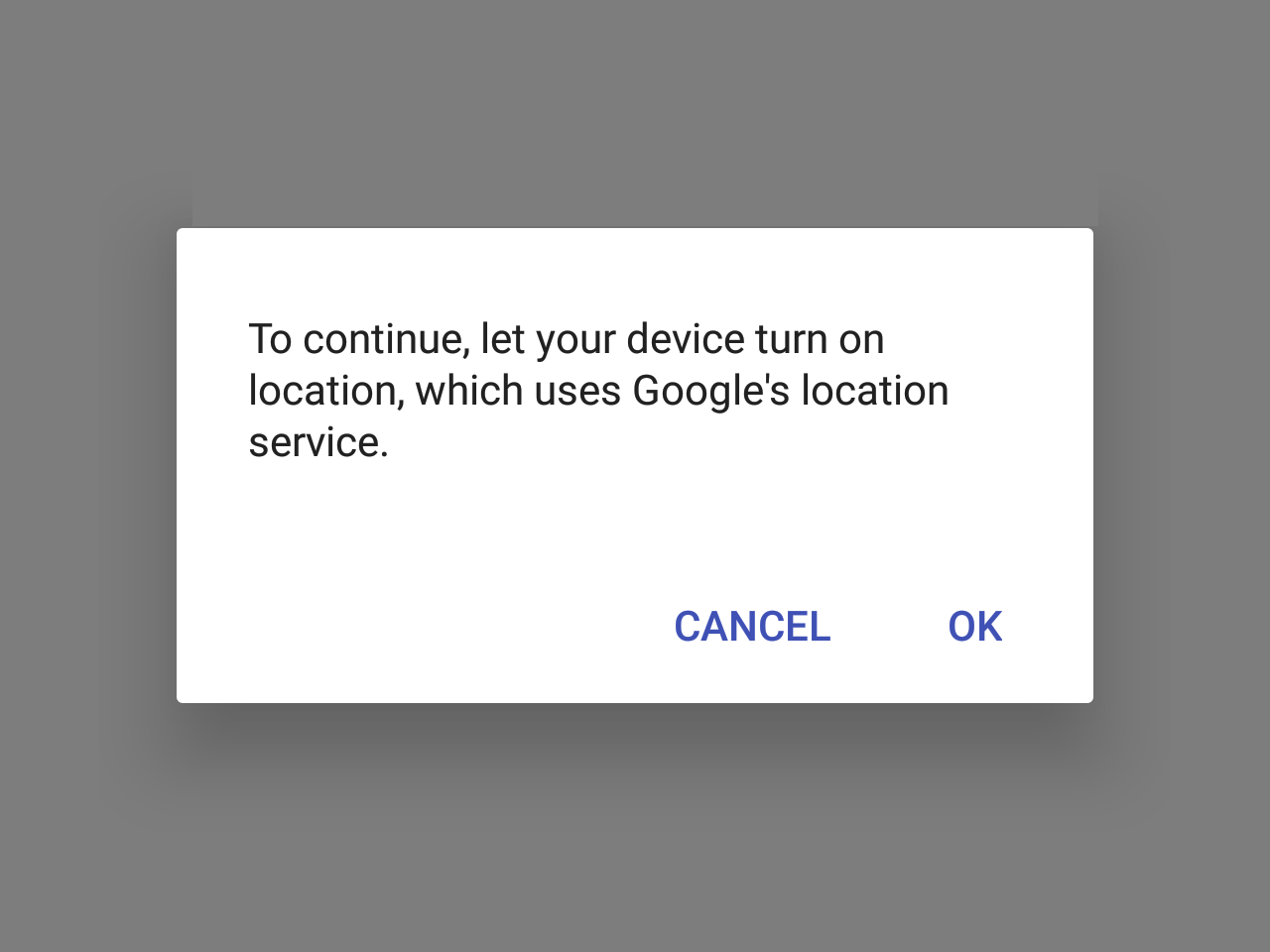
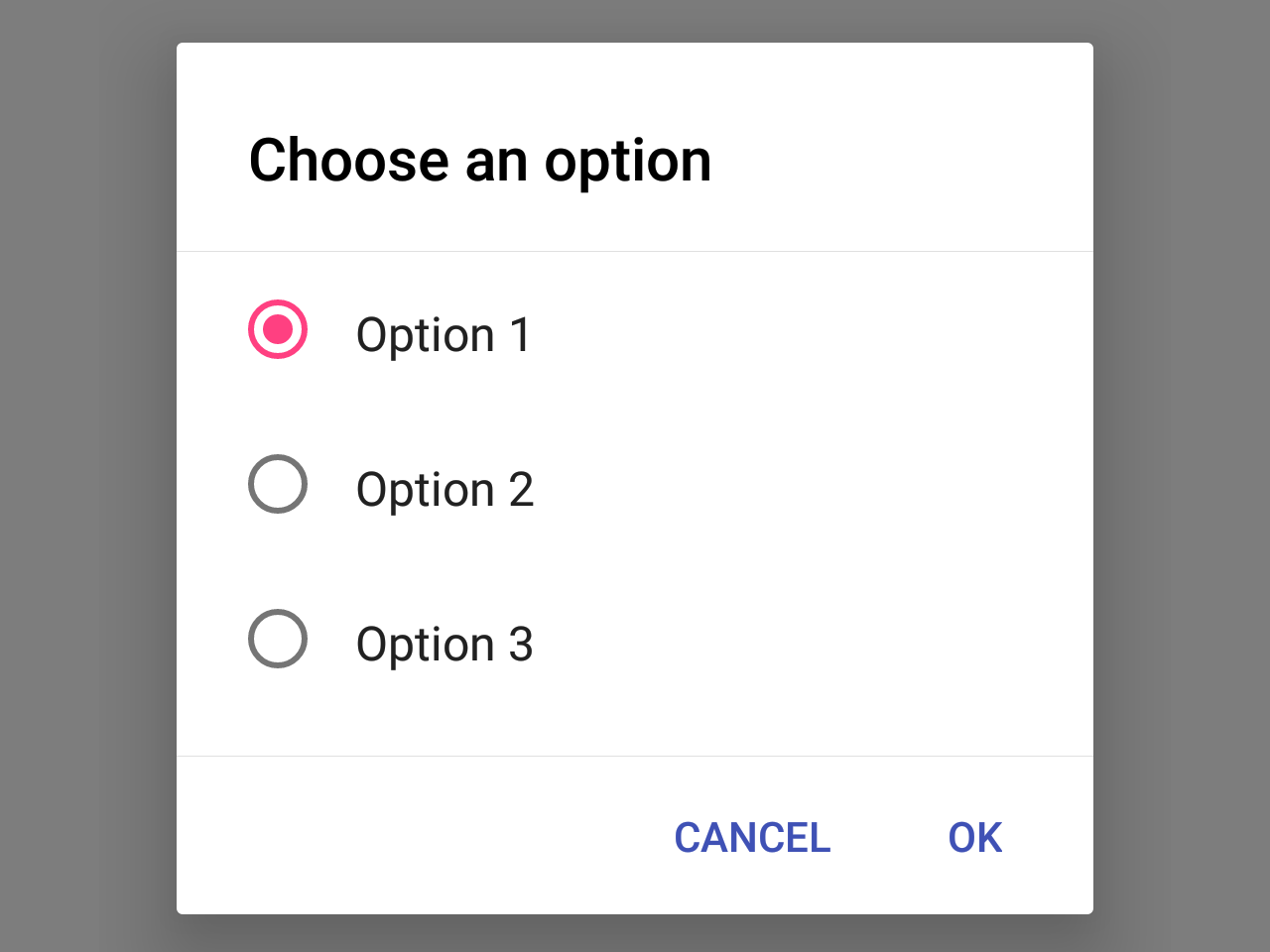
Usage
import * as React from 'react';
import { View } from 'react-native';
import { Button, Paragraph, Dialog, Portal, Provider } from 'react-native-paper';
const MyComponent = () => {
const [visible, setVisible] = React.useState(false);
const showDialog = () => setVisible(true);
const hideDialog = () => setVisible(false);
return (
<Provider>
<View>
<Button onPress={showDialog}>Show Dialog</Button>
<Portal>
<Dialog visible={visible} onDismiss={hideDialog}>
<Dialog.Title>Alert</Dialog.Title>
<Dialog.Content>
<Paragraph>This is simple dialog</Paragraph>
</Dialog.Content>
<Dialog.Actions>
<Button onPress={hideDialog}>Done</Button>
</Dialog.Actions>
</Dialog>
</Portal>
</View>
</Provider>
);
};
export default MyComponent;
Props
dismissable
Type:
boolean
Default value:
true
Determines whether clicking outside the dialog dismiss it.
style
Type:
StyleProp<ViewStyle>
theme
Type:
ReactNativePaper.Theme