Menu.Item
A component to show a single list item inside a Menu.
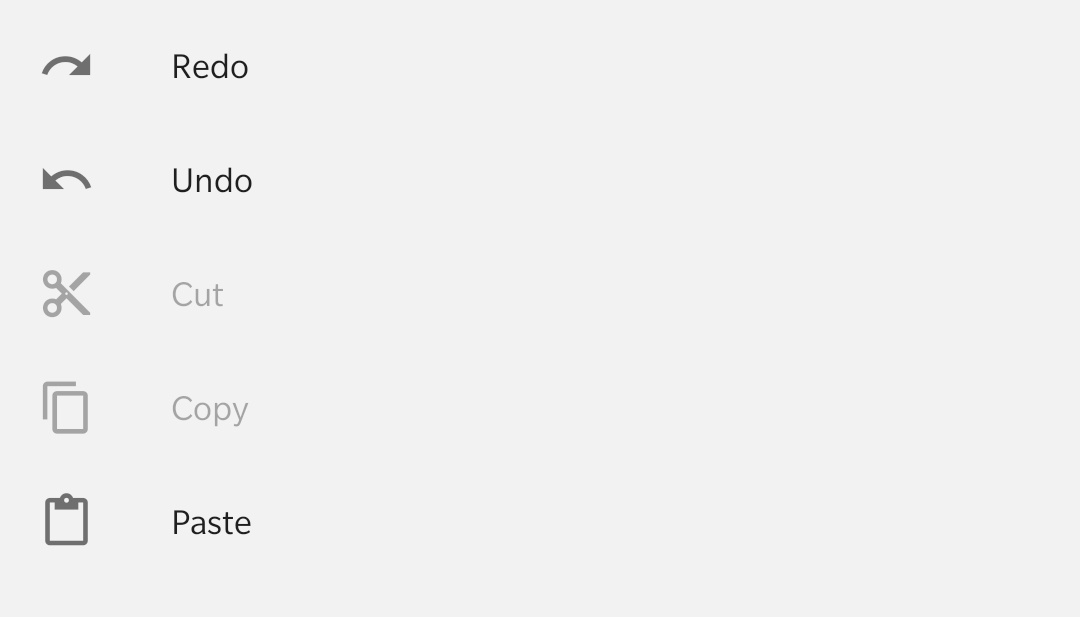
Usage
import * as React from 'react';
import { View } from 'react-native';
import { Menu } from 'react-native-paper';
const MyComponent = () => (
<View style={{ flex: 1 }}>
<Menu.Item icon="redo" onPress={() => {}} title="Redo" />
<Menu.Item icon="undo" onPress={() => {}} title="Undo" />
<Menu.Item icon="content-cut" onPress={() => {}} title="Cut" disabled />
<Menu.Item icon="content-copy" onPress={() => {}} title="Copy" disabled />
<Menu.Item icon="content-paste" onPress={() => {}} title="Paste" />
</View>
);
export default MyComponent;
Props
disabled
Type:
boolean
Whether the 'item' is disabled. A disabled 'item' is greyed out and onPress
is not called on touch.
style
Type:
StyleProp<ViewStyle>
contentStyle
Type:
StyleProp<ViewStyle>
titleStyle
Type:
StyleProp<TextStyle>
theme
Type:
ReactNativePaper.Theme
accessibilityLabel
Type:
string
Accessibility label for the Touchable. This is read by the screen reader when the user taps the component.