List.Item
A component to show tiles inside a List.
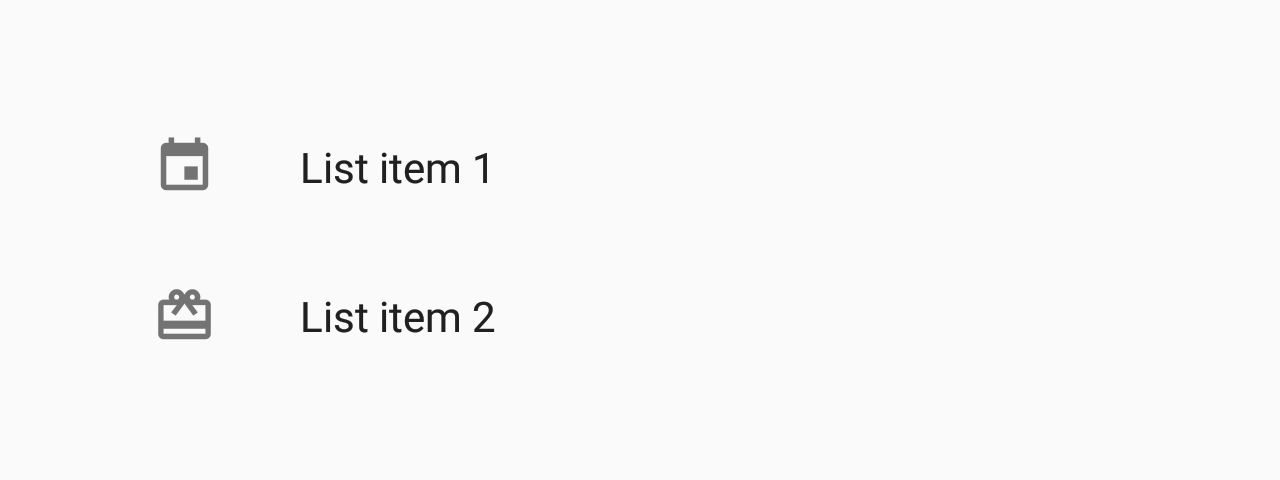
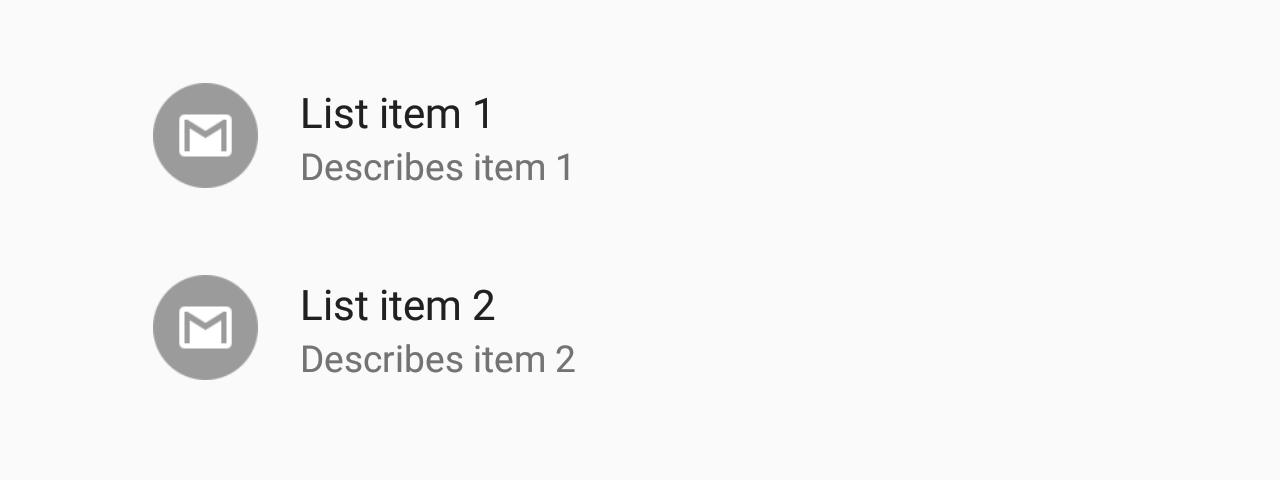
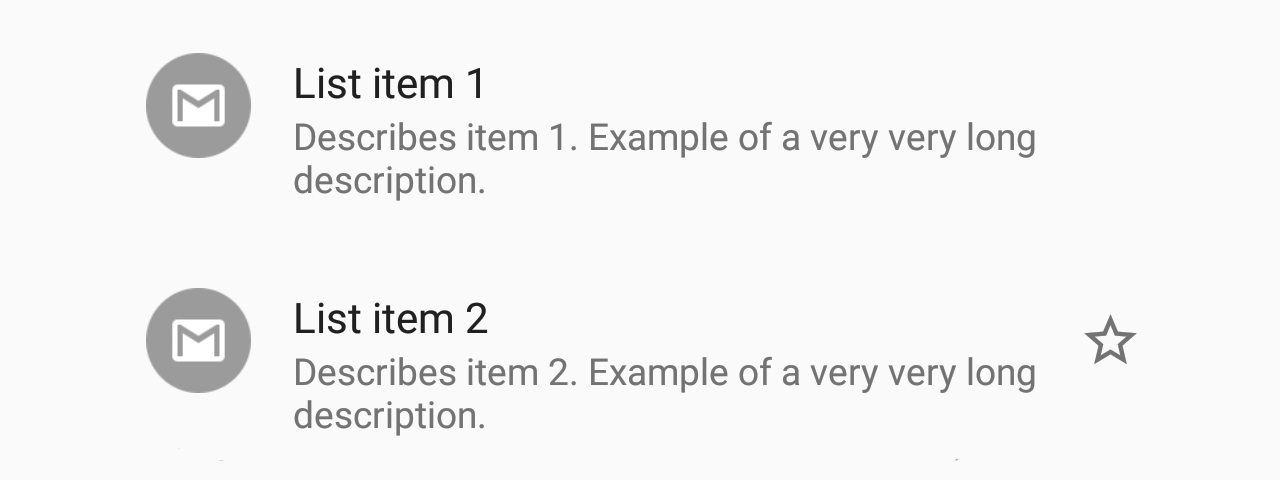
Usage
import * as React from 'react';
import { List } from 'react-native-paper';
const MyComponent = () => (
<List.Item
title="First Item"
description="Item description"
left={props => <List.Icon {...props} icon="folder" />}
/>
);
export default MyComponent;
Props
title
(required)Type:
React.ReactNode
| ((props: {
selectable: boolean;
ellipsizeMode: EllipsizeProp | undefined;
color: string;
fontSize: number;
}) => React.ReactNode)
Title text for the list item.
description
Type:
React.ReactNode
| ((props: {
selectable: boolean;
ellipsizeMode: EllipsizeProp | undefined;
color: string;
fontSize: number;
}) => React.ReactNode)
Description text for the list item or callback which returns a React element to display the description.
left
Type:
(props: {
color: string;
style: {
marginLeft: number;
marginRight: number;
marginVertical?: number;
};
}) => React.ReactNode
Callback which returns a React element to display on the left side.
right
Type:
(props: {
color: string;
style?: {
marginRight: number;
marginVertical?: number;
};
}) => React.ReactNode
Callback which returns a React element to display on the right side.
theme
Type:
ReactNativePaper.Theme
titleNumberOfLines
Type:
number
Default value:
1
Truncate Title text such that the total number of lines does not exceed this number.
descriptionNumberOfLines
Type:
number
Default value:
2
Truncate Description text such that the total number of lines does not exceed this number.
titleEllipsizeMode
Type:
EllipsizeProp
Ellipsize Mode for the Title. One of 'head'
, 'middle'
, 'tail'
, 'clip'
.
See ellipsizeMode
descriptionEllipsizeMode
Type:
EllipsizeProp
Ellipsize Mode for the Description. One of 'head'
, 'middle'
, 'tail'
, 'clip'
.
See ellipsizeMode
...TouchableRipple props
Edit this page