Searchbar
Searchbar is a simple input box where users can type search queries.
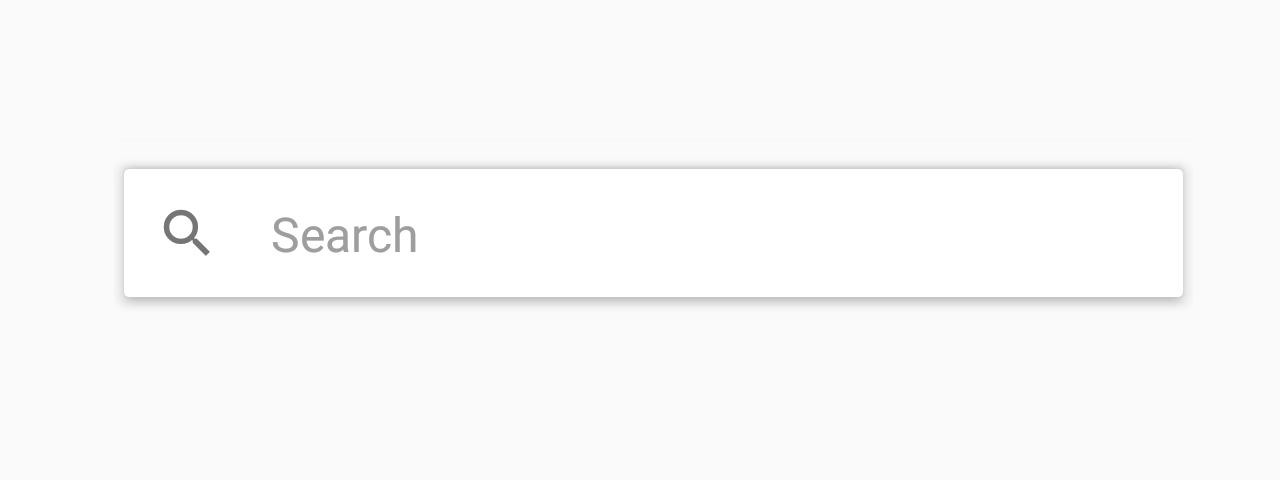
Usage
import * as React from 'react';
import { Searchbar } from 'react-native-paper';
const MyComponent = () => {
const [searchQuery, setSearchQuery] = React.useState('');
const onChangeSearch = query => setSearchQuery(query);
return (
<Searchbar
placeholder="Search"
onChangeText={onChangeSearch}
value={searchQuery}
/>
);
};
export default MyComponent;
Props
clearAccessibilityLabel
Type:
string
Default value:
'clear'
Accessibility label for the button. This is read by the screen reader when the user taps the button.
searchAccessibilityLabel
Type:
string
Default value:
'search'
Accessibility label for the button. This is read by the screen reader when the user taps the button.
onChangeText
Type:
(query: string) => void
Callback that is called when the text input's text changes.
style
Type:
StyleProp<ViewStyle>
theme
Type:
ReactNativePaper.Theme