TextInput.Icon
A component to render a leading / trailing icon in the TextInput
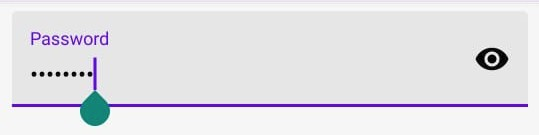
Usage
import * as React from 'react';
import { TextInput } from 'react-native-paper';
const MyComponent = () => {
const [text, setText] = React.useState('');
return (
<TextInput
label="Password"
secureTextEntry
right={<TextInput.Icon name="eye" />}
/>
);
};
export default MyComponent;
Props
color
Type:
((isTextInputFocused: boolean) => string | undefined) | string
Color of the icon or a function receiving a boolean indicating whether the TextInput is focused and returning the color.
style
Type:
StyleProp<ViewStyle>
theme
Type:
ReactNativePaper.Theme